Thursday, November 29, 2007
Basics of C for Technical Editors
# include
main ( )
{
printf ("hello, world\n");
}
All C programs starts with the execution of the function main. All C programs must include a function with the name main.
The round brackets or parentheses means that main is a function.
The empty brackets mean that the function main expects no arguments.
The statements of the function are enclosed in curly brackets or braces.
C statements are expression statements: an expression followed by a semicolon. For example,
i = 0; or i = i + 1;
printf prints the characters within the quotes on the screen. Thes characters "hello, world\n" is called a string or character string or string constant.
Strings are always put between inverted commas.
A semicolon after characters indicates that it is a statement.
"n' is the escape sequence, because when ENTER is pressed it goes to the next line and not a new line or paragraph.
VARIABLES: In C, there are two main types of variablesC: numeric variables that hold only numbers or values, and string variables that hold text, from one to several characters long.
Al variables are declared first. The declaration consists of the variable type name and a list of variables. For example,
int fahr, celsius
This declares the variables fahr and celsius.
A variable can also declared with an initializer that consists of an equals sign (=) and an expression, that is usually a constant.
Note: Characters must always be put between single quotes.
Compared to variables, value of a Constant never changes.
OPERATORS: Operators are symbols used to tell the computer to do a certain mathematical or logical manipulation. For more, read this tutorial on Operators.
Statements: C statements are in the form of expressions followed by a semicolon. For example,
i = 0; or i = i + 1;
Boolean expressions checks true or false.
ARRAYS: Array is a variable that can hold more than one value. For example, int a[10]; declares an array, named a, consisting of ten elements.
This is how a fucntion will look like in C:
Return-type function name (argument declaration){
declarations and statements
}
POINTERS: Pointers point to a memory address or a variable that stores a memory address. For an editor, the key to locate a pointer is the absence or presence of the asterik or *. A pointer declaration will have the form,
Sunday, November 11, 2007
Different levels of computer programming
The different levels of computer programming are:
1. First generation-Language the computer can obey instantly. In the form of 0's and 1's. The command tells the computer what to do and the operand tells the computer where to find and store the data.
2. Second generation-Also called Assembly language. In this language 0's and 1's are replaced by symbolic codes written by humans. This include mnemonic codes and symbolic addresses. The instructions are of 3 parts;Label or tag (symbols defined by coders), OP code (code that tells the computer what to do) and Operand (tells the computer where to store and locate information).
3. Third- Also called High-level languages-More procedure and problem solving oriented. Language is written using alphabets, numbers and special characters. Rules called Syntax is developed. Eg: C, Fortran, COBOL, Java.
4. Fourth-Non-procedural, Very High-Level language. Applications can bew created and information easily gathered. Eg: IBM's Query, MS Office, MS Visual Studio.
5. Fifth-Also called Natural language.
Notes: All Source programs must be translated using a Translator to machine language called Object program.
Assemblers converts Assembly language to machine language.
Interpreter translates high-level language to object code, compiler converts high-level source code to object programs.
Newspaper front pages - June 5
Some images of front pages of newspapers after votes were counted on June 4, 2024 after a ridiculously long parliament elections. Did the ...
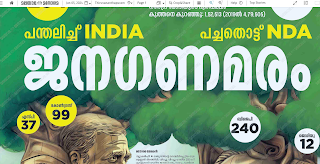
-
Some images of front pages of newspapers after votes were counted on June 4, 2024 after a ridiculously long parliament elections. Did the ...
-
In the 70s and 80s, school principals used to instruct students to read English newspapers such as The Hindu for improving English skills. B...
-
On October 27, 2021, the supreme court of India ordered that an independent expert committee will investigate the revelations made in the P...